In this chapter we will see to create an event in Yii. To show events in action, we need data.
Preparing the DB
Step 1 − Create a new database. Database can be prepared in the following two ways.
- In the terminal run mysql -u root –p
- Create a new database via CREATE DATABASE helloworld CHARACTER SET utf8 COLLATE utf8_general_ci;
Step 2 − Configure the database connection in the config/db.php file. The following configuration is for the system used currently.
<?php
return [
'class' => 'yii\db\Connection',
'dsn' => 'mysql:host=localhost;dbname=helloworld',
'username' => 'vladimir',
'password' => '12345',
'charset' => 'utf8',
];
?>
Step 3 − Inside the root folder run ./yii migrate/create test_table. This command will create a database migration for managing our DB. The migration file should appear in the migrations folder of the project root.
Step 4 − Modify the migration file (m160106_163154_test_table.php in this case) this way.
<?php
use yii\db\Schema;
use yii\db\Migration;
class m160106_163154_test_table extends Migration {
public function safeUp() {
$this->createTable("user", [
"id" => Schema::TYPE_PK,
"name" => Schema::TYPE_STRING,
"email" => Schema::TYPE_STRING,
]);
$this->batchInsert("user", ["name", "email"], [
["User1", "[email protected]"],
["User2", "[email protected]"],
["User3", "[email protected]"],
["User4", "[email protected]"],
["User5", "[email protected]"],
["User6", "[email protected]"],
["User7", "[email protected]"],
["User8", "[email protected]"],
["User9", "[email protected]"],
["User10", "[email protected]"],
["User11", "[email protected]"],
]);
}
public function safeDown() {
$this->dropTable('user');
}
}
?>
The above migration creates a user table with these fields: id, name, and email. It also adds a few demo users.
Step 5 − Inside the project root run ./yii migrate to apply the migration to the database.
Step 6 − Now, we need to create a model for our user table. For the sake of simplicity, we are going to use the Gii code generation tool. Open up this url: http://localhost:8080/index.php?r=gii. Then, click the “Start” button under the “Model generator” header. Fill in the Table Name (“user”) and the Model Class (“MyUser”), click the “Preview” button and finally, click the “Generate” button.
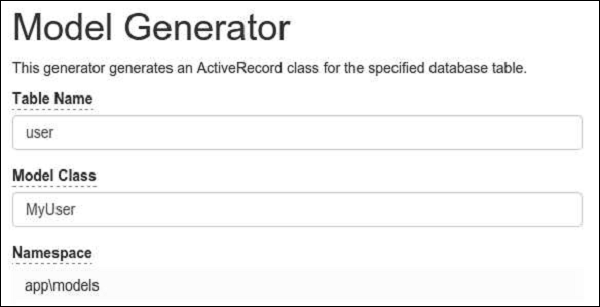
The MyUser model should appear in the models directory.
Create an Event
Assume we want to send an email to the admin whenever a new user registers on our web site.
Step 1 − Modify the models/MyUser.php file this way.
<?php
namespace app\models;
use Yii;
/**
* This is the model class for table "user".
*
* @property integer $id
* @property string $name
* @property string $email
*/
class MyUser extends \yii\db\ActiveRecord {
const EVENT_NEW_USER = 'new-user';
public function init() {
// first parameter is the name of the event and second is the handler.
$this->on(self::EVENT_NEW_USER, [$this, 'sendMailToAdmin']);
}
/**
* @inheritdoc
*/
public static function tableName() {
return 'user';
}
/**
* @inheritdoc
*/
public function rules() {
return [
[['name', 'email'], 'string', 'max' => 255]
];
}
/**
* @inheritdoc
*/
public function attributeLabels() {
return [
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
];
}
public function sendMailToAdmin($event) {
echo 'mail sent to admin using the event';
}
}
?>
In the above code, we define a “new-user” event. Then, in the init() method we attach the sendMailToAdmin function to the “new-user” event. Now, we need to trigger this event.
Step 2 − Create a method called actionTestEvent in the SiteController.
public function actionTestEvent() {
$model = new MyUser();
$model->name = "John";
$model->email = "[email protected]";
if($model->save()) {
$model->trigger(MyUser::EVENT_NEW_USER);
}
}
In the above code, we create a new user and trigger the “new-user” event.
Step 3 − Now type http://localhost:8080/index.php?r=site/test-event, you will see the following.

Leave a Reply